The observer pattern is used in situation when one object is updated, a series of objects need to be notified. For example, a teacher uses a homework assigner to assign homework to students. whenever the homework assigner is updated, the registered student accounts will be notified.
Java has made this more convenient for it has already created an Observer interface and an Observable class.
//Create the HomeworkAssigner
public class HomeworkAssigner extends Observable {
private JTextArea textArea;
private String text;
public HomeworkAssigner() {
textArea = new JTextArea(5, 50);
textArea.setEditable(true);
text = "";
textArea.addKeyListener(new HomeworkListener());
}
private class HomeworkListener extends KeyAdapter {
@Override
public void keyReleased(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_ENTER) {
System.out.println("listener fired");
String newText = textArea.getText();
if (!newText.equals(text)) {
text = newText;
//Observable methods to notify Observers
setChanged();
notifyObservers();
}
}
}
}
public String getText() {
return text;
}
public JTextArea getTextArea() {
return textArea;
}
}
//Create StudentAccount
public class StudentAccount implements Observer {
private String name;
private String emailAddress;
public StudentAccount(String n, String e) {
name = n;
emailAddress = e;
}
@Override
public void update (Observable nwa, Object arg) {
String text = ((HomeworkAssigner)nwa).getText();
//send email to student
System.out.println("email sent to "+name+": "+text);
}
}
//The runner
public class TestDemo {
public static void main(String[] args) {
HomeworkAssigner nwa = new HomeworkAssigner();
Observer ob1 = new StudentAccount("Maria", "maria@gmail.com");
Observer ob2 = new StudentAccount("John", "john@yahoo.com");
nwa.addObserver(ob1);
nwa.addObserver(ob2);
JFrame frame = new JFrame();
frame.getContentPane().add(nwa.getTextArea());
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setVisible(true);
}
}
----------------------------------------------------------------------------------------------------------------
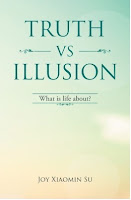
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
Java has made this more convenient for it has already created an Observer interface and an Observable class.
//Create the HomeworkAssigner
public class HomeworkAssigner extends Observable {
private JTextArea textArea;
private String text;
public HomeworkAssigner() {
textArea = new JTextArea(5, 50);
textArea.setEditable(true);
text = "";
textArea.addKeyListener(new HomeworkListener());
}
private class HomeworkListener extends KeyAdapter {
@Override
public void keyReleased(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_ENTER) {
System.out.println("listener fired");
String newText = textArea.getText();
if (!newText.equals(text)) {
text = newText;
//Observable methods to notify Observers
setChanged();
notifyObservers();
}
}
}
}
public String getText() {
return text;
}
public JTextArea getTextArea() {
return textArea;
}
}
public class StudentAccount implements Observer {
private String name;
private String emailAddress;
public StudentAccount(String n, String e) {
name = n;
emailAddress = e;
}
@Override
public void update (Observable nwa, Object arg) {
String text = ((HomeworkAssigner)nwa).getText();
//send email to student
System.out.println("email sent to "+name+": "+text);
}
}
//The runner
public class TestDemo {
public static void main(String[] args) {
HomeworkAssigner nwa = new HomeworkAssigner();
Observer ob1 = new StudentAccount("Maria", "maria@gmail.com");
Observer ob2 = new StudentAccount("John", "john@yahoo.com");
nwa.addObserver(ob1);
nwa.addObserver(ob2);
JFrame frame = new JFrame();
frame.getContentPane().add(nwa.getTextArea());
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setVisible(true);
}
}
----------------------------------------------------------------------------------------------------------------
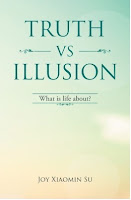
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment