Defined by Java, addNotify makes a JFrame displayable by connecting it to a native screen resource. Making a frame displayable will cause any of its children to be made displayable. This method is called internally by the toolkit and should not be called directly by programs. And for a JComponent, addNotify notifies this component that it now has a parent component. When this method is invoked, the chain of parent components is set up with
In the following sample code, a JPanel p2 is set as the content pane of the JFrame. Another JPanel p1 is added to p2, and a JTextField tf is added to p1. When you execute the code, the addNotify is called in the sequence of JFrame, p2, p1, and tf. If you comment out the super.addNotify() in the JFrame, the whole gui will not show at all. If you comment out the super.addNotify() in any of the other components, you are not able to type anything in the text field.
import java.awt.BorderLayout;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class AddNotifyTest extends JFrame {
public AddNotifyTest() {
// System.out.println("Contructing.....");
JPanel p1 = new JPanel() {
@Override
public void addNotify() {
System.out.println("p1....");
super.addNotify();
}
};
JPanel p2 = new JPanel() {
@Override
public void addNotify() {
System.out.println("p2....");
super.addNotify();
}
};
JTextField tf = new JTextField (20) {
@Override
public void addNotify() {
System.out.println("tf....");
super.addNotify();
}
};
p1.setLayout(new BorderLayout());
p2.setLayout(new BorderLayout());
p1.add(tf);
p2.add(p1);
setContentPane(p2);
tf.addKeyListener(new KeyAdapter() {
@Override
public void keyPressed(KeyEvent e){
if (e.getKeyCode() == KeyEvent.VK_F1){
System.out.println("F1 pressed....");
}
}
});
setDefaultCloseOperation(DISPOSE_ON_CLOSE);
setSize(300, 200);
}
@Override
public void addNotify() {
System.out.println("JFrame....");
super.addNotify();
}
public static void main(String[] args){
javax.swing.SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
AddNotifyTest at = new AddNotifyTest();
at.setVisible(true);
}
});
}
}
----------------------------------------------------------------------------------------------------------------
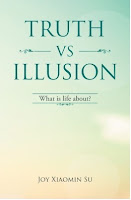
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
KeyboardAction
event listeners. This method is called by the toolkit internally and should not be called directly by programs.In the following sample code, a JPanel p2 is set as the content pane of the JFrame. Another JPanel p1 is added to p2, and a JTextField tf is added to p1. When you execute the code, the addNotify is called in the sequence of JFrame, p2, p1, and tf. If you comment out the super.addNotify() in the JFrame, the whole gui will not show at all. If you comment out the super.addNotify() in any of the other components, you are not able to type anything in the text field.
import java.awt.BorderLayout;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class AddNotifyTest extends JFrame {
public AddNotifyTest() {
// System.out.println("Contructing.....");
JPanel p1 = new JPanel() {
@Override
public void addNotify() {
System.out.println("p1....");
super.addNotify();
}
};
JPanel p2 = new JPanel() {
@Override
public void addNotify() {
System.out.println("p2....");
super.addNotify();
}
};
JTextField tf = new JTextField (20) {
@Override
public void addNotify() {
System.out.println("tf....");
super.addNotify();
}
};
p1.setLayout(new BorderLayout());
p2.setLayout(new BorderLayout());
p1.add(tf);
p2.add(p1);
setContentPane(p2);
tf.addKeyListener(new KeyAdapter() {
@Override
public void keyPressed(KeyEvent e){
if (e.getKeyCode() == KeyEvent.VK_F1){
System.out.println("F1 pressed....");
}
}
});
setDefaultCloseOperation(DISPOSE_ON_CLOSE);
setSize(300, 200);
}
@Override
public void addNotify() {
System.out.println("JFrame....");
super.addNotify();
}
public static void main(String[] args){
javax.swing.SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
AddNotifyTest at = new AddNotifyTest();
at.setVisible(true);
}
});
}
}
----------------------------------------------------------------------------------------------------------------
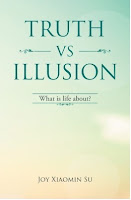
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment