The proxy pattern is used to cash an object that is expensive to create (e.g. loading large media file) or retrieve from remote site through network. It acts as the interface for accessing the object. One of its major characters is to create/retrieve the object on the fly.
For example, a company has an office and need to access it to get information frequently.
//The interface
public interface Office {
public Integer getDailySale();
public void setDailyGoal();
public boolean isOverSea();
}
//The concrete class
public class OverSeaOffice implements Office {
private String manager;
private boolean overSea;
public OverSeaOffice(String m) {
manager = m;
overSea = true;
}
public Integer getDailySale() {
return new Integer(500);
}
public void setDailyGoal() {
System.out.println("Today is to make 200 contracts");
}
public boolean isOverSea() {
return overSea;
}
}
//The proxy. Only need to create the OverSeaOffice once
public class OfficeProxy implements Office {
private String manager;
private Office theOffice;
public OfficeProxy(String m) {
manager = m;
}
public Integer getDailySale() {
if (theOffice == null) {
theOffice = new OverSeaOffice(manager);
}
return theOffice.getDailySale();
}
public void setDailyGoal() {
if (theOffice == null) {
theOffice = new OverSeaOffice(manager);
}
theOffice.setDailyGoal();
}
public boolean isOverSea() {
if (theOffice == null) {
theOffice = new OverSeaOffice(manager);
}
return theOffice.isOverSea();
}
}
//The runner
public class OfficeRunner {
public static void main(String[] args) {
OfficeProxy op = new OfficeProxy("Smith");
System.out.println(op.getDailySale());
op.setDailyGoal();
System.out.println("Is the office oversea? " + op.isOverSea());
}
}
-------------------------------------------------------------------------------------------------------------
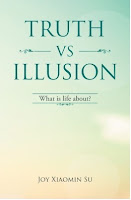
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
For example, a company has an office and need to access it to get information frequently.
//The interface
public interface Office {
public Integer getDailySale();
public void setDailyGoal();
public boolean isOverSea();
}
//The concrete class
public class OverSeaOffice implements Office {
private String manager;
private boolean overSea;
public OverSeaOffice(String m) {
manager = m;
overSea = true;
}
public Integer getDailySale() {
return new Integer(500);
}
public void setDailyGoal() {
System.out.println("Today is to make 200 contracts");
}
public boolean isOverSea() {
return overSea;
}
}
//The proxy. Only need to create the OverSeaOffice once
public class OfficeProxy implements Office {
private String manager;
private Office theOffice;
public OfficeProxy(String m) {
manager = m;
}
public Integer getDailySale() {
if (theOffice == null) {
theOffice = new OverSeaOffice(manager);
}
return theOffice.getDailySale();
}
public void setDailyGoal() {
if (theOffice == null) {
theOffice = new OverSeaOffice(manager);
}
theOffice.setDailyGoal();
}
public boolean isOverSea() {
if (theOffice == null) {
theOffice = new OverSeaOffice(manager);
}
return theOffice.isOverSea();
}
}
//The runner
public class OfficeRunner {
public static void main(String[] args) {
OfficeProxy op = new OfficeProxy("Smith");
System.out.println(op.getDailySale());
op.setDailyGoal();
System.out.println("Is the office oversea? " + op.isOverSea());
}
}
-------------------------------------------------------------------------------------------------------------
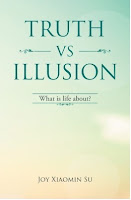
If you have ever asked yourself these questions, this is the book for you. What is the meaning of life? Why do people suffer? What is in control of my life? Why is life the way it is? How can I stop suffering and be happy? How can I have a successful life? How can I have a life I like to have? How can I be the person I like to be? How can I be wiser and smarter? How can I have good and harmonious relations with others? Why do people meditate to achieve enlightenment? What is the true meaning of spiritual practice? Why all beings are one? Read the book free here.
No comments:
Post a Comment